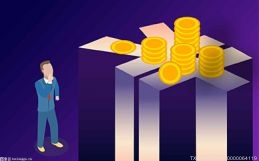
下面是一个使用 Spring Cloud Bus 和 RabbitMQ 的完整示例。在此示例中,我们将创建两个服务:Config Service 和 Client Service。Config Service 负责存储应用程序的配置文件,Client Service 则使用这些配置文件来配置自身。
配置 Config Service
在 Config Service 中,我们需要将配置文件存储在 Git 存储库中,并启用 Spring Cloud Bus 和 RabbitMQ 支持。
首先,可以在 application.yml 文件中添加以下配置:
(资料图)
spring: cloud: config: server: git: uri: https://github.com/your-git-repo/config-repo.git bus: enabled: true trace: enabled: true rabbit: enabled: true
在这个示例中,我们将 Config Service 配置为从 GitHub 存储库中加载应用程序的配置文件接下来,需要在 Config Service 中添加一个 REST 控制器,该控制器可以将 Spring Cloud Bus 消息发送到 RabbitMQ。可以使用以下代码来实现:
@RestControllerpublic class ConfigController { private final BusRefreshListener busRefreshListener; @Autowired public ConfigController(BusRefreshListener busRefreshListener) { this.busRefreshListener = busRefreshListener; } @PostMapping("/refresh") public void refresh() { busRefreshListener.refresh(); }}
在这个示例中,我们创建了一个 REST 控制器,该控制器将在 /refresh 路径上监听 POST 请求。当接收到该请求时,控制器将调用 BusRefreshListener bean 的 refresh() 方法,该方法将向 Spring Cloud Bus 发送一个刷新消息。
最后,我们需要在 Config Service 中添加一个 BusRefreshListener bean,该 bean 将在收到 Spring Cloud Bus 消息时触发配置文件的重新加载。可以使用以下代码来实现:
@Componentpublic class BusRefreshListener implements ApplicationListener { private final ConfigurableApplicationContext context; @Autowired public BusRefreshListener(ConfigurableApplicationContext context) { this.context = context; } @Override public void onApplicationEvent(RefreshRemoteApplicationEvent event) { context.refresh(); } public void refresh() { context.publishEvent(new RefreshRemoteApplicationEvent(this, "", "")); }}
在这个示例中,我们创建了一个 BusRefreshListener bean,该 bean 实现了 ApplicationListener 接口,并在收到 RefreshRemoteApplicationEvent 事件时触发了应用程序上下文的刷新。我们还添加了一个 refresh() 方法,该方法将创建一个新的 RefreshRemoteApplicationEvent 事件,并将其发布到应用程序上下文中。
配置 Client Service
在 Client Service 中,我们需要添加一个依赖于 Config Service 的组件,并在收到 Spring Cloud Bus 消息时重新加载配置文件。
可以在 application.yml 文件中添加以下配置:
spring: cloud: config: uri: http://localhost:8888 name: client-service bus: enabled: true trace: enabled: true rabbit: enabled: true
在这个示例中,我们将 Client Service 配置为使用 Config Service 中存储的配置文件。我们还启用了 Spring Cloud Bus 和 RabbitMQ 支持。
最后,我们需要在 Client Service 中添加一个 RefreshScope bean,该 bean 将在收到 Spring Cloud Bus 消息时重新加载应用程序的配置文件。可以使用以下代码来实现:
@Component@RefreshScopepublic class ConfigComponent { @Value("${message:Hello World!}") private String message; public String getMessage() { return message; }}
在这个示例中,我们创建了一个 ConfigComponent bean,该 bean 带有一个 @RefreshScope 注解,以便它可以在收到 Spring Cloud Bus 消息时重新加载。我们还将一个名为 message 的属性注入到该 bean 中,并在 getMessage() 方法中返回该属性的值。